Google Shopping - Outputting Sale Price Effective Date with PHP
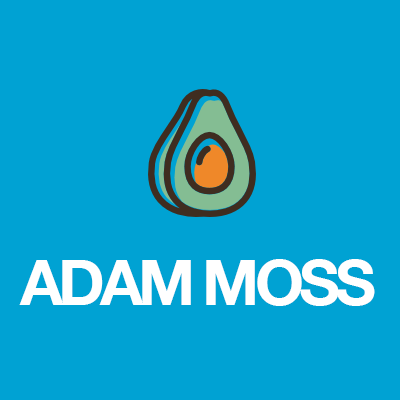
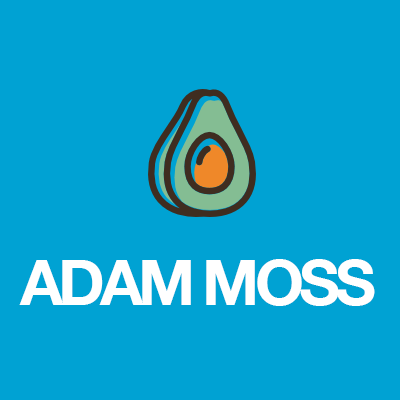
In a recent project I was building a good ol’ fashioned Google Shopping feed, something I’ve done more times than I’ve had hot dinners, except this time I encountered a new attribute called sale_price_effective_date
After a swift read of Google’s documentation I realised it wasn’t as straightforward as other PHP dates I have outputted in the past. There’s a timezone… and a random T right in the middle, and there’s a sale FROM and TO date!!
Ultimately, Google wants something like this:
2011-03-01T16:00-0800/2011-03-03T16:00-0800
As a Magento user I’ll show you the function I used to get to this hideous string of data. I’ve stripped it down slightly for simplicity.
<?php
public function getSalePriceEffectiveDate($product)
{
// return if no sale price
if ($product->getSpecialPrice() <= 0) {
return false;
}
$currentTimeString = strtotime("now");
$saleFromTimeString = $product->getSpecialFromDate()
? strtotime($product->getSpecialFromDate())
: $currentTimeString;
$saleToTimeString = $product->getSpecialToDate()
? strtotime($product->getSpecialToDate())
: false;
if ($saleToTimeString) {
$from = $this->googleDate($saleFromTimeString);
$to = $this->googleDate($saleToTimeString);
return $from."/".$to;
}
return false;
}
public function googleDate($timeString)
{
return date("Y-m-d\TH:iO", $timeString);
}
The key part here is how each date is formatted based on Google’s requirements:
date("Y-m-d\TH:iO", $saleFromTimeString)
The \T
is what allows the T to intersect the date and time, and the O
outputs the timezone difference in hours between the server location and GMT.
Another sneaky part of the script is how I’ve set the $saleFromTimeString
as the current time in case one hasn’t been set. If there’s an end date then surely there has to be a start date right?
It’s all relatively simple but if you’re not familiar with all aspects of the PHP date()
function it can be tricky! Hopefully this comes in useful for someone.