Magento 2 Basics Part 1 - Setting Up Your Module
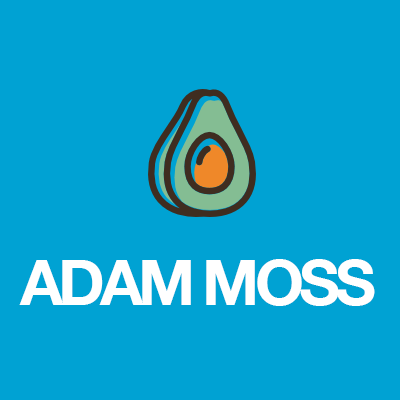
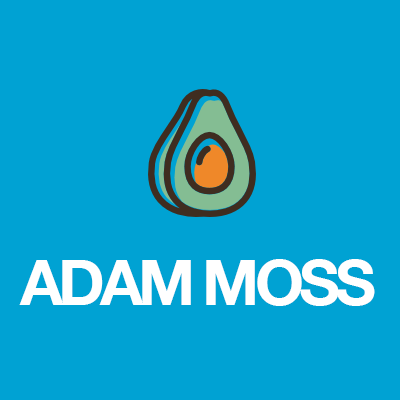
In the following tutorials I’m going to take you through the very basics of creating a simple, local Magento 2 module. The series will cover the following topics:
- Part 1 - Setting up your module
- Part 2 - Creating a frontend controller
- Part 3 - Creating a helper
- Part 4 - Creating an observer
- Part 5 - Creating an admin page
- Part 6 - Using plugins
Note - I’m going to cover the basics of a local module created in app/code. Modules should ideally be developed in separate repositories and installed with Composer.
Let’s start by clearing up exactly what a module is. Magento defines a module as the following:
A module is basically a package of code that encapsulates a particular business feature or set of features.
Magento 2 encourages modules to take responsibility for their own separate features and to explicity declare any dependancies (if any). The module should be working code in its own right and if possible be unaware of the system it is being plugged into.
With that in mind, let’s go through the steps of creating our own module.
Step 1 - Decide on Namespace (Vendor) and Module Name
Just like in Magento 1 a module must belong to a namespace, which is now referred to as a vendor:
- Vendor: Magefox
- Module: Example
With this in mind create the following directory: app/code/Magefox/Example
Step 2 - Create Magefox/Example/etc/module.xml
This file is basically the same as your module declaration file in Magento 1 (the one in app/etc/modules), except it is now conveniently stored within your module. Everything in one place!
<config xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:noNamespaceSchemaLocation="urn:magento:framework:Module/etc/module.xsd">
<module name="Magefox_Example" setup_version="1.0.0">
</module>
</config>
Step 3 - Create Magefox/Example/registration.php
This file was introduced shortly before GA was released and simply serves as a way to register your module as a component with Magento. Simply add your vendor/module name as the second argument.
<?php
\Magento\Framework\Component\ComponentRegistrar::register(
\Magento\Framework\Component\ComponentRegistrar::MODULE,
'Magefox_Example',
__DIR__
);
All that’s left for us to do is to activate our module, so using command lin navigate to your Magento 2 root directory and run the following command:
php bin/magento setup:upgrade
Congratulations you’ve just created a Magento 2 module! If you want to check it has installed properly login to the admin and go to Stores > Configuration > Advanced > Advanced and you should see it in the “Disable Modules Output” section.